What is Wordnet?
WordNet is a large lexical database for words in the English language. It provides synonyms, definitions, and other useful information and relations between synonyms.
Wordnet groups English words into sets of synonyms called Synsets. It can be seen as a semantic database used to find synonyms, antonyms, part of speech information and related words in English.
Wordnet has been used extensively in computational linguistics, cognitive psychology, and machine translation research.
What are Synonyms in NLP?
Synonyms are words that have the same or similar meaning. They can be used in different contexts and with different meanings but they still have the same underlying meaning. Synonyms are a great way to find new words if you don’t know how to express what you want to say.
In some cases, the meaning of synonymous words is not always interchangeable, but they often share some of their intentions.
for example:
The word “regretful” is synonymous with “bad” but can’t be interchanged in the sentence below.
“A bad motor caused the ship to sink”
WordNet Python Examples using NLTK: Find Synonyms and Antonyms from NLTK
The purpose of this tutorial is to show you how you can use NLTK WordNet in Python to find synonyms and antonyms.
NLTK is a natural language processing library with a built-in WordNet database. So, in this tutorial, we will write a simple python code that will help us find synonyms for any given word by using NLTK WordNet in Python.
Import WordNet
from nltk.corpus import wordnet
A Synset is a WordNet synonym set. It is a collection of words that have the same meaning, and are grouped together in a sense as they are related to each other.
Synset python example:
from nltk.corpus import wordnet
synset = wordnet.synsets("cunning")
print(synset)
Output:

From the results, we can see that the word “cunning” is synonymous with “craft”, “crafty” and “clever”.
To see more words in the synset, we can expand and loop through the synset lists for more words.
for syns in synset:
for word in syns.lemmas():
print(word.name())
Output:
craft
craftiness
cunning
foxiness
guile
slyness
wiliness
cunning
cunning
cute
crafty
cunning
dodgy
foxy
guileful
knavish
slick
sly
tricksy
tricky
wily
clever
cunning
ingenious
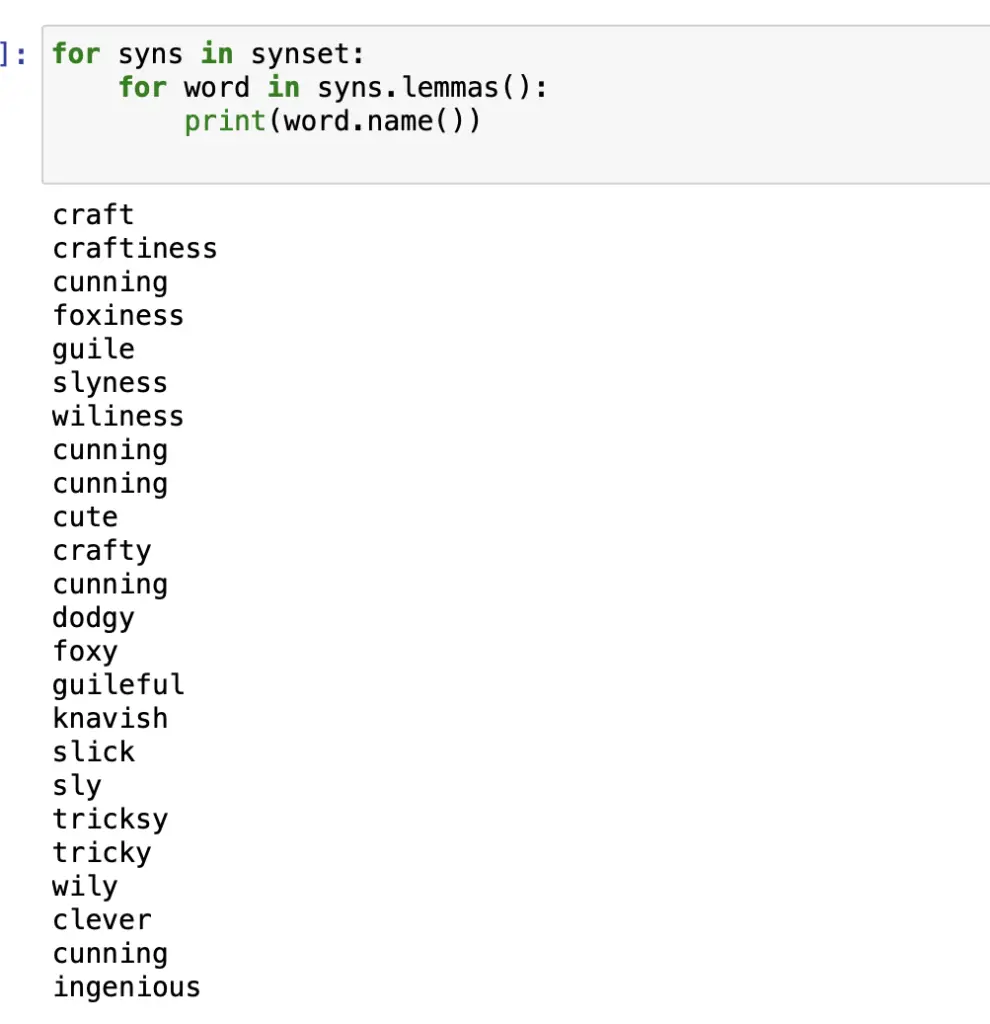
What are Antonyms in NLP?
Antonyms are words that have opposite or nearly opposite meanings. They are usually opposites in the way they are used. For example, “close” is an antonym of “open”.
They are also known as antonym pairs.
Some examples of antonyms are:
- Hot and cold
- Loud and quiet
- Up and down
- In and out
- Night and day
How to find the Antonym of a word
synset = wordnet.synsets("fear")
for syns in synset:
for word in syns.lemmas():
if word.antonyms():
print(word.antonyms())
Output:
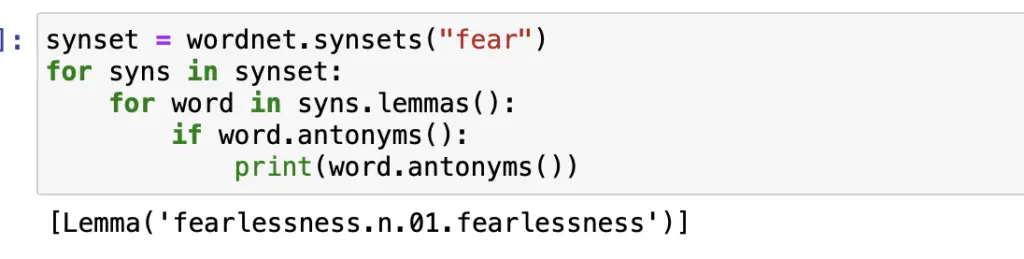
We can write a python function that outputs both the synonym and antonyms of any given word
from nltk.corpus import wordnet
from nltk.tokenize import word_tokenize
def synonym_antonyms_parser(sentence):
tokens = word_tokenize(sentence)
for token in tokens:
for syns in wordnet.synsets(token):
for word in syns.lemmas():
synonym = word.name()
if word.antonyms():
antonym = word.antonyms()
print( "Word: {} Synonym: {} Antonym: {}".format(token, synonym, antonym))
sentence = "good is bad"
synonym_antonyms_parser(sentence)
Output:
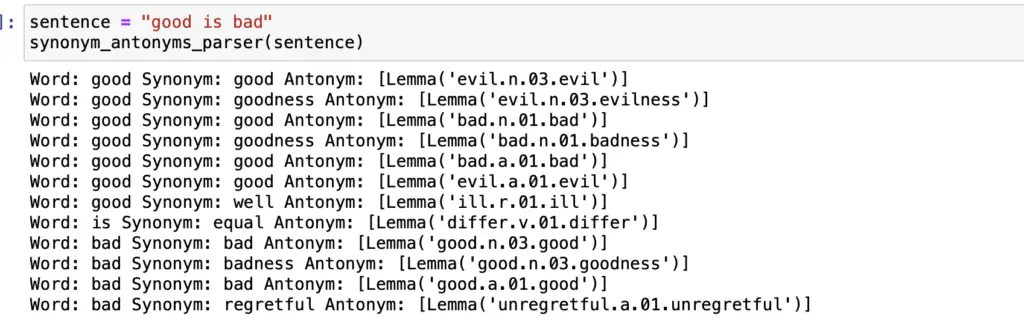
There are many words in the English language that do not have antonyms. Proper Nouns, Material Nouns, Proper Adjectives, Adjectives of Number are some categories that can never have antonyms. Words like “clothe” or “American” can’t be opposed by an antonym.
What are Hypernyms in NLP?
A hypernym is a broader word that encompasses more specific words. Hypernyms are words that have a more general meaning than other words. For example, the words “dog” and “cat” are hyponyms of the word “animal.”
Another good example of a hypernym is the word “book.” The word book is related to words like “library,” “reading,” and “writing.”
print ("Hypernym for 'cat': ", wordnet.synset('cat.n.01').hypernyms())
From the output, we can see that “cat” is a hyponym of “feline”. Feline is a family of mammals in the order Carnivora, informally referred to as cats.

print ("Hypernym for 'fish': ", wordnet.synset('fish.n.01').hypernyms())
What is the hyponym of fish? From the output we can see that the hypernym for fish is “aquatic vertebrate”

Hypernymy and hyponymy are useful in NLP for analyzing content to provide the most relevant response possible for a question and answering system.
What are Holonyms in NLP?
Holonyms are words that share the same root word but have different meanings.
An example of a holonym is “bank.” The word “bank” can refer to a financial institution, or an edge of land that goes down to the water and protects what’s behind it from flooding, or a place where you put money to earn interest.
What are Meronyms in NLP?
Meronyms are a part of the word that is used to refer to a whole object or its properties.
For example: The word “car” is a meronym for “wheels”, “doors”, and “engine”.
In the sentence “the trunk of a tree“, the word “trunk” is the meronym of “tree.”
Noun meronyms include: arm, leg, wing. Adjective meronyms include: right-handed, left-handed
car = wordnet.synset('car.n.01')
print(car.part_meronyms())
Output:
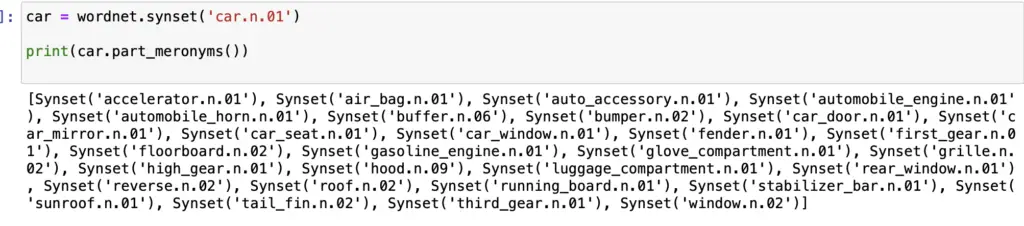
Is WordNet a taxonomy?
Yes, WordNet can be considered a taxonomy or a type of lexical database that organizes words and their meanings into a network of interrelated concepts.
Words are organized into synsets (sets of synonyms) based on their meanings and relationships. Each synset represents a distinct concept or idea, and is linked to other synsets through semantic relationships such as hyponymy (is-a), hypernymy (has-a), meronymy (part-of), and holonymy (member-of).
This hierarchical structure of synsets and their relationships can be seen as a taxonomy of concepts and their interrelationships. For example, the synset for “dog” is a hyponym of the synset for “animal”, which is a hyponym of the synset for “living thing”, and so on.
In addition to its use as a taxonomy, WordNet is also widely used for natural language processing tasks such as word sense disambiguation, information retrieval, and text classification
Further Reading on Natural Language Processing
- Get started with Natural Language Processing
- Morphological segmentation
- Word segmentation
- Parsing
- Parts of speech tagging
- breaking sentence
- Named entity recognition (NER)
- Natural language generation
- Word sense disambiguation
- Deep Learning (Recurrent Neural Networks)
- WordNet
- Language Modeling
Interested in learning how to build for production? check out my publication TreapAI.com